About Razor PDF:
https://www.nuget.org/packages/RazorPDF
Example(Creating PDF with ASP.Net MVC):
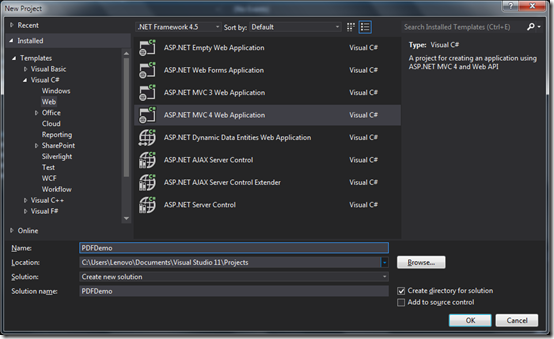
Once you click on OK. It will ask for type of project. We are going to create ASP.Net MVC internet application.
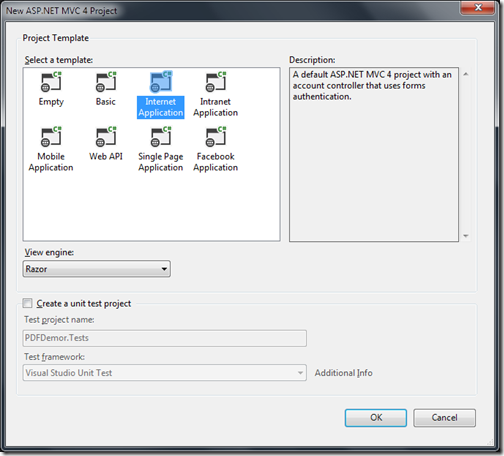
Once you click on it will create an application. The next thing you need to install a NuGet package. You need to type following command on your NuGet Package manager console.

Like following.
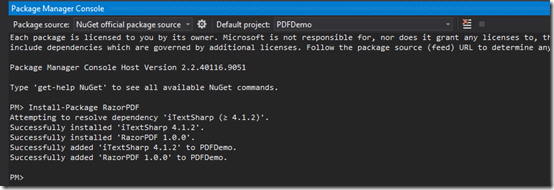
Now our application is ready to create PDF files. Now to create an example Let’s create a model class ‘Customer’ to create a listing of customers in the application.
namespace PDFDemor.Models { public class Customer { public int CustomerID { get ; set ; } public string FirstName { get ; set ; } public string LastName { get ; set ; } } } |
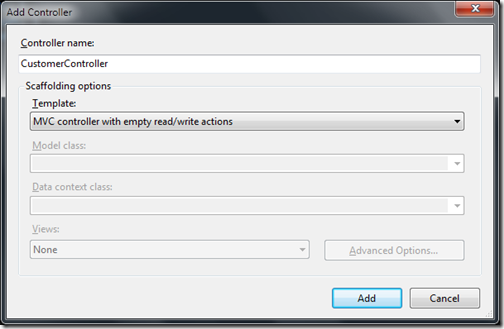
Now once you click Add It will create CustomerController. In index ActionResult I have created following code. Where I have created an list and pass it to view.
using System; using System.Collections.Generic; using System.Linq; using System.Web; using System.Web.Mvc; using PDFDemo.Models; namespace PDFDemo.Controllers { public class CustomerController : Controller { // // GET: /Customer/ public ActionResult Index() { List<Customer> customers= new List<Customer>(); for ( int i = 1; i <= 10; i++) { Customer customer = new Customer { CustomerID = i, FirstName = string .Format( "FirstName{0}" , i.ToString()), LastName = string .Format( "LastName{0}" , i.ToString()) }; customers.Add(customer); } return View(customers); } } } |
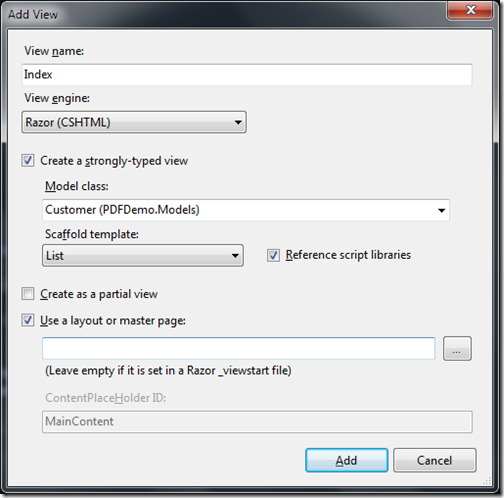
Once you click add it will create a view and now let’s run that application. It will look like following.
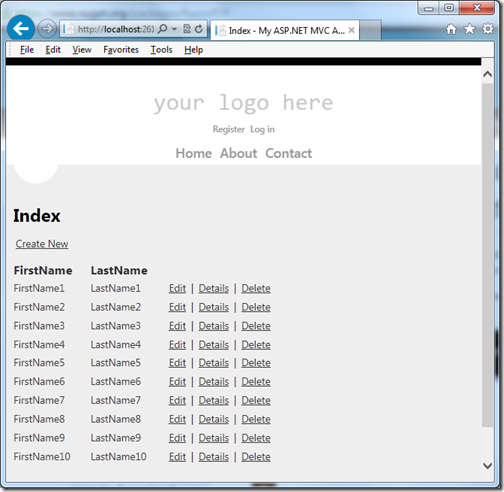
So everything looks good now. Now It’s time to create PDF document for same list. Let’s create a new action result method called PDF in same controller.
public ActionResult PDF() { List<Customer> customers = new List<Customer>(); for ( int i = 1; i <= 10; i++) { Customer customer = new Customer { CustomerID = i, FirstName = string .Format( "FirstName{0}" , i.ToString()), LastName = string .Format( "LastName{0}" , i.ToString()) }; customers.Add(customer); } return new RazorPDF.PdfResult(customers, "PDF" ); } |
@model List< PDFDemo.Models.Customer > @{ Layout = null; } <!DOCTYPE html> < html > < head > </ head > < body > < h2 >Html List in PDF</ h2 > < table width = "100%" > < tr > < td >First Name</ td > < td >Last Name</ td > </ tr > @foreach (var item in Model) { < tr > < td >@item.FirstName</ td > < td >@item.LastName</ td > </ tr > } </ table > </ body > </ html > |
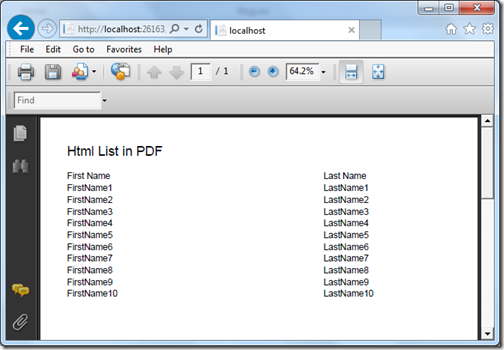
That’s it. It’s very easy to create PDF with ASP.Net with Razor PDF.
No comments :
Post a Comment